반응형
'Error Lens'라는 Extension을 추천하더라. 에러를 잘 설명해주더라.
추출하고 싶은 위젯에 커서를 올려서 Code Action을 살펴보면 'Extract Widget'이 있다.
이 것을 이용하면 자동으로 위젯을 따로 빼서 만들어준다. 개사기이것을 직접 만들어보는 것으로 하자.
widget 폴더를 새로 만들어서 button.dart 파일을 만들자.
class Button extends StatelessWidget {
final String text;
final Color bgcolor;
final Color textColor;
const Button({
// key는 나중에 설명할 것이다.
super.key,
required this.text,
required this.bgcolor,
required this.textColor,
});
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
color: bgcolor,
borderRadius: BorderRadius.circular(45),
),
child: Padding(
padding: const EdgeInsets.symmetric(
vertical: 20,
horizontal: 40,
),
child: Text(
text,
style: TextStyle(
fontSize: 20,
color: textColor,
),
),
),
);
}
}
사실 statelessWidget만 치면 자동완성으로 알아서 해주지만, 직접 코딩 해보자....class 만들고 StatelessWidget을 상속해 오는거다.
그리고 text, bgcolor, textColor를 입력받을 수 있도록 선언한 후에 build는 자동완성을 이용하고, 안에 있는 Container는 이전에 만들어 뒀던 것을 가져온다.
하드코딩 되어 있는 값들을 직접 변수로 수정하는 것을 잊지 말자.
그리고, super.key는 나중에 설명해준다고 한다.
import './widgets/button.dart';
const Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Button(
text: 'Transfer',
bgcolor: Color(0xFFF2B33A),
textColor: Colors.black,
),
Button(
text: 'Request',
bgcolor: Color(0xFF1F2123),
textColor: Colors.white,
),
],
),
이렇게 간편하게 불러올 수 있다. 참고로, spaceBetween을 이용하면 최대한 공간을 넓게 쓰도록 만들어 준다.
그 결과는 다음 사진과 같다.
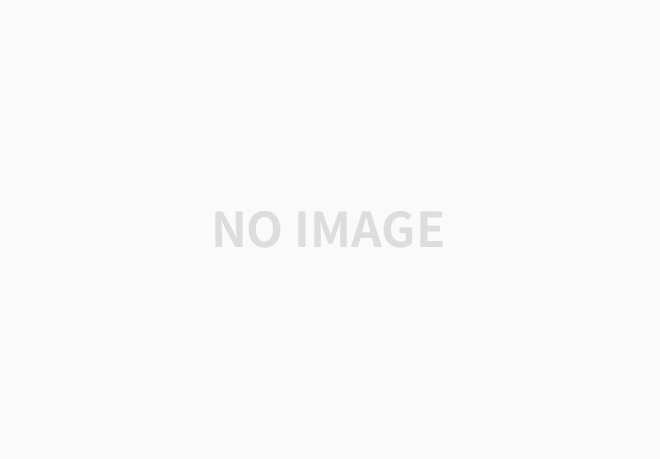
전체 코드:
main.dart
import 'package:flutter/material.dart';
import './widgets/button.dart';
void main() {
runApp(const App());
}
class App extends StatelessWidget {
const App({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: const Color(0xFF181818),
body: Padding(
padding: const EdgeInsets.symmetric(
horizontal: 20,
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
// SizedBox: 빈 박스
const SizedBox(
height: 80,
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
children: [
Column(
crossAxisAlignment: CrossAxisAlignment.end,
children: [
const Text(
'Hey, Selena',
style: TextStyle(
color: Colors.white,
fontSize: 28,
fontWeight: FontWeight.w800,
),
),
Text(
'Welcome back',
style: TextStyle(
color: Colors.white.withOpacity(0.8),
fontSize: 18,
),
),
],
),
],
),
const SizedBox(
height: 120,
),
Text(
'Total balance',
style: TextStyle(
fontSize: 22,
color: Colors.white.withOpacity(0.8),
),
),
const SizedBox(
height: 5,
),
const Text(
// dart에서 $는 변수를 가져올때 사용하므로 \를 붙여줘야 한다.
'\$5 194 482',
style: TextStyle(
fontSize: 48,
fontWeight: FontWeight.w600,
color: Colors.white,
),
),
const SizedBox(
height: 30,
),
const Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Button(
text: 'Transfer',
bgcolor: Color(0xFFF2B33A),
textColor: Colors.black,
),
Button(
text: 'Request',
bgcolor: Color(0xFF1F2123),
textColor: Colors.white,
),
],
),
],
),
),
),
);
}
}
./widgets/button.dart
import 'package:flutter/material.dart';
class Button extends StatelessWidget {
final String text;
final Color bgcolor;
final Color textColor;
const Button({
// key는 나중에 설명할 것이다.
super.key,
required this.text,
required this.bgcolor,
required this.textColor,
});
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
color: bgcolor,
borderRadius: BorderRadius.circular(45),
),
child: Padding(
padding: const EdgeInsets.symmetric(
vertical: 20,
horizontal: 40,
),
child: Text(
text,
style: TextStyle(
fontSize: 20,
color: textColor,
),
),
),
);
}
}
반응형
'앱 만들기 프로젝트 > Flutter' 카테고리의 다른 글
Flutter - 3.7 Icons and Transform (0) | 2024.10.25 |
---|---|
Flutter - 3.6 Cards (1) | 2024.10.25 |
Flutter - 3.4 Code Actions (0) | 2024.10.25 |
Flutter - 3.3 VSCode Settings (1) | 2024.10.25 |
Flutter - 3.2 Buttons Section (0) | 2024.10.25 |